Table Of Content
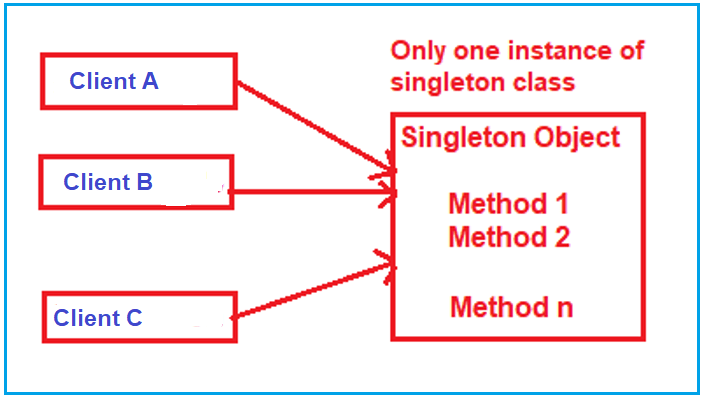
We increment the instanceCounter variable whenever an instance of the Singleton class is created, so the best place to increment and print it is constructor. So, whenever we create an instance of singleton or invoke the PrintDetails() method of singleton, we end up creating multiple objects. Now, Imagine a situation where we need to invoke PrintDetails() from different classes of a given project. So, what we need to do is create multiple objects of this SingletonImp class.
Thread-safe Singleton
Thread Safe Singleton without using locks and no lazy instantiation. Pentalog Connect is your free pass to a large community of top engineers who excel in developing outstanding and impactful digital products. When joining, you receive access to a wealth of resources that will feed your appetite for quality content and your need for professional growth. Our content helps you to learn technologies easily and quickly for learners of all levels. By accessing this platform, you acknowledge that you have reviewed and consented to abide by our Terms of Use and Privacy Policy, designed to safeguard your experience and privacy rights.
Sign up or log in
For this reason, the singleton pattern is sometimes considered an antipattern or pattern singleton. We may look to a Load Balancer for an example of the Singleton design pattern. Because servers may dynamically come online and offline, only a single instance of the class can be created, and every request must pass through the single object that knows the status of a web farm. The above VoteMachine is a singleton class with the static constructor.
Real-life Singleton Class
85 Must-Know C# Interview Questions and Answers [2024] - Simplilearn
85 Must-Know C# Interview Questions and Answers .
Posted: Mon, 15 Apr 2024 07:00:00 GMT [source]
Regardless of the personal identities of the individuals who form governments, the title, “The Government of X”, is a global point of access that identifies the group of people in charge. Nowadays, the Singleton pattern has become so popular that people may call something a singleton even if it solves just one of the listed problems. Give everything a proper prefix and now you can use my_singleton_method() in lieu of my_singleton.method(). In addition to the other discussion here, it may be worth noting that you can have global-ness, without limiting usage to one instance.
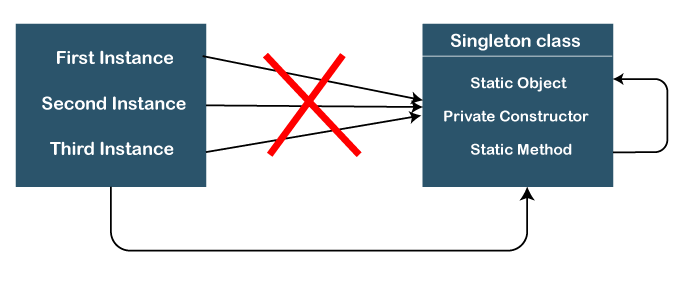
Now, we proved that it's not necessary to create a new instance of an object for common methods like PrintDetails(). In the above-mentioned source code we created a private static readonly object type variable and initialized it. Then in the SingleInstance property we wrapped the code of instance creation under the lock, so that one thread can enter the code at a time and other thread waits until first thread finishes its execution.
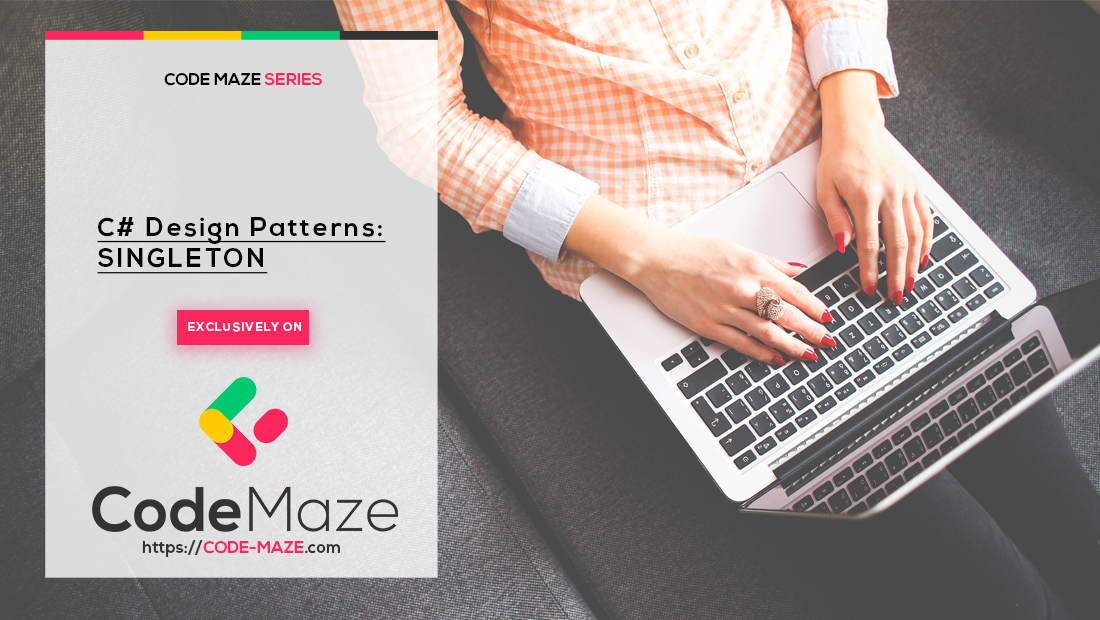
Code source of the full example available on hnrck/singleton_example. Use Ctrl+Left/Right to switch messages, Ctrl+Up/Down to switch threads, Ctrl+Shift+Left/Right to switch pages. Here, I have created a Console Application using C#, as shown below. This C++11 implementation is based on the pre C++98 implementation in the book [citation needed].
Using this appraoch, the double-checked locking works just fine. The singleton is used when you need to have the same object to perform specific actions.The singleton is also very often used in the implementation of other design patterns. Even though its more secure and complete than first one, still there is a minor drawback in this implementation. So, in the next example we can see how to implement a complete and secure Singleton design pattern. As you can see on the result, we got the exact same instance even though we create two instances of that class.
Disadvantages of the Singleton Pattern in C++ Design Patterns
I hope you understand why we need the Singleton Design Pattern in C# with Examples. Note that you can always adjust this limitation and allow creating any number of Singleton instances. The only piece of code that needs changing is the body of the getInstance method. Especially if it grabs any system resources that aren't automatically released on process exit.
Categories of Design Patterns
Singleton is a creational design pattern that lets you ensure that a class has only one instance, while providing a global access point to this instance. If you wonder why would we need only one instance of a class (one object of a class), the most common reason for this is to control access to some shared resource, such as a database or a file. However, this design pattern considers as one of the most abused design pattern, due to some developers use it without proper understanding where to apply this pattern in their program. The Singleton Design Pattern is a Creational pattern, and it is one of the simplest design pattern in gang of four. The main functionality of this pattern is, to create only one instance of a class and to provide only one global access point to that object within the container (JVM; in case of Java).
It will get torn down and deallocated when the program terminates, which is the normal, desired behavior for a singleton. We can use lock over an object if any thread tries to access the instance and in that case, the other thread waits until the lock is released. Here I have created a method called PrintDetails() with a parameter called message and displayed it. As of now, we have not implemented any singleton design principle in this class. A better solution is to make the class itself responsible for keeping track of its sole instance.
Use the Singleton pattern when a class in your program should have just a single instance available to all clients; for example, a single database object shared by different parts of the program. In this example, the database connection class acts as a Singleton. This class doesn’t have a public constructor, so the only way to get its object is to call the getInstance method.
The static constructor runs only once per app domain when any static member of a class is accessed. The Singleton pattern is useful when we need to have only one instance of a class, for example, a single database connection shared by multiple objects as creating a separate database connection for every object may be costly. In the following code, the thread is locked on a shared object and checks whether an instance has been created or not with double checking.
This static member ensures that memory is allocated only once, preserving the single instance of the Singleton class. A singleton is a class which only allows one instance of itself to be created - and gives simple, easy access to said instance. A singleton design pattern is all about ensuring that a class has only one instance in the application. It is a design pattern from the Creational Pattern of Gang of Four (GOF) Design Patterns. You can create a singleton class by using the static constructor.
No comments:
Post a Comment